Table of Contents
ToggleUltrasonic sensors are a staple in robotics, automation, and various electronics projects due to their ability to detect and measure distance without contact. This blog will explain how ultrasonic sensors work and guide you through setting one up with an Arduino to create a basic distance measurement system.
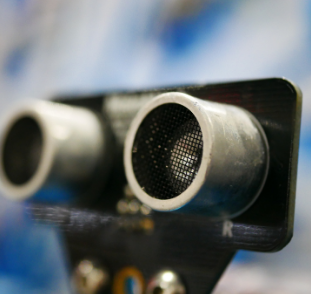
Understanding ultrasonic sensors
An ultrasonic sensor works by sending an ultrasonic sound wave (a frequency higher than humans can hear) to an object and then listening for the echo of that wave. By calculating the time between sending the signal and receiving the echo, the sensor can determine the distance to an object. This method is similar to how bats navigate in the dark.
The Principle of Ultrasonic Sensing
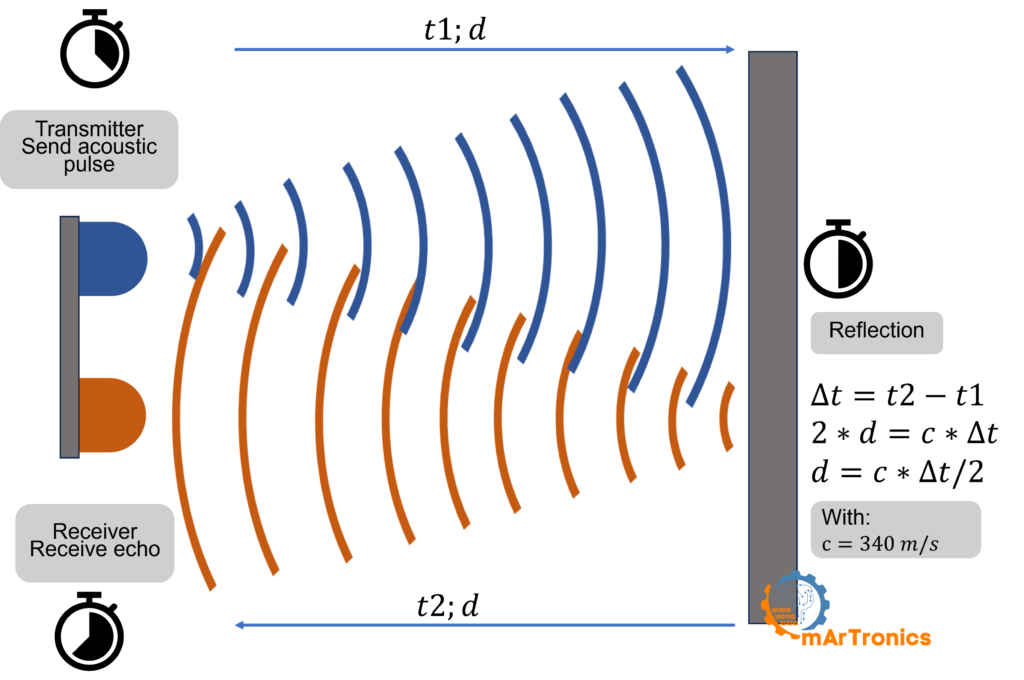
An ultrasonic sensor typically comprises two main components: a transmitter and a receiver. The transmitter emits high-frequency sound waves, which travel through the air until they encounter an object. Once these sound waves hit the object, they reflect back towards the sensor, where the receiver detects them as an echo.
- Transmission of Sound Waves: The transmitter side of the sensor emits an acoustic pulse, a sound wave at a frequency that is generally above the human hearing range, usually around 40 kHz. This sound wave spreads out in a cone-shaped manner from the sensor.
- Reflection of Sound Waves: Once the emitted sound wave encounters an object, it bounces back towards the sensor. This returned signal is often referred to as the echo. The nature of the reflected sound wave can be influenced by the shape, size, and material of the object it encounters.
- Reception of Echo: The receiver part of the sensor then detects this echo. By precisely timing how long it takes for the echo to return, the sensor can calculate the distance to the object. This calculation is based on the speed of sound in air, which is approximately 340 meters per second under standard conditions.
- Distance Calculation: The distance to the object is typically calculated by measuring the time interval between when the sound wave was emitted and when the echo was received. Using the formula
Distance =1/2×Time×Speed of Sound, the sensor computes the distance. The factor of 1/2 is used because the sound wave travels to the object and then back to the sensor, hence covering the distance twice.
The most common ultrasonic sensor used in DIY projects is the HC-SR04. This sensor offers excellent distance detection, is very affordable and is widely available. The HC-SR04 can measure distances from 2cm to 400cm with an accuracy of up to 3mm, making it suitable for a wide range of application
Components needed
Arduino Uno: The microcontroller board that processes the data from the ultrasonic sensor.
HC-SR04 ultrasonic sensor: This sensor will detect the distance.
Jumper wires: Used to connect the sensor to the Arduino.
Breadboard: To make connections easier and more organised.
Electronic configuration and wiring
Connecting the HC-SR04 to the Arduino
Connect the VCC pin of the HC-SR04 to the 5V output of the Arduino.
GND pin goes to one of the GND pins on the Arduino.
Trig pin should be connected to a digital I/O pin on the Arduino, such as D2.
Echo pin must be connected to another digital I/O pin, for example D3
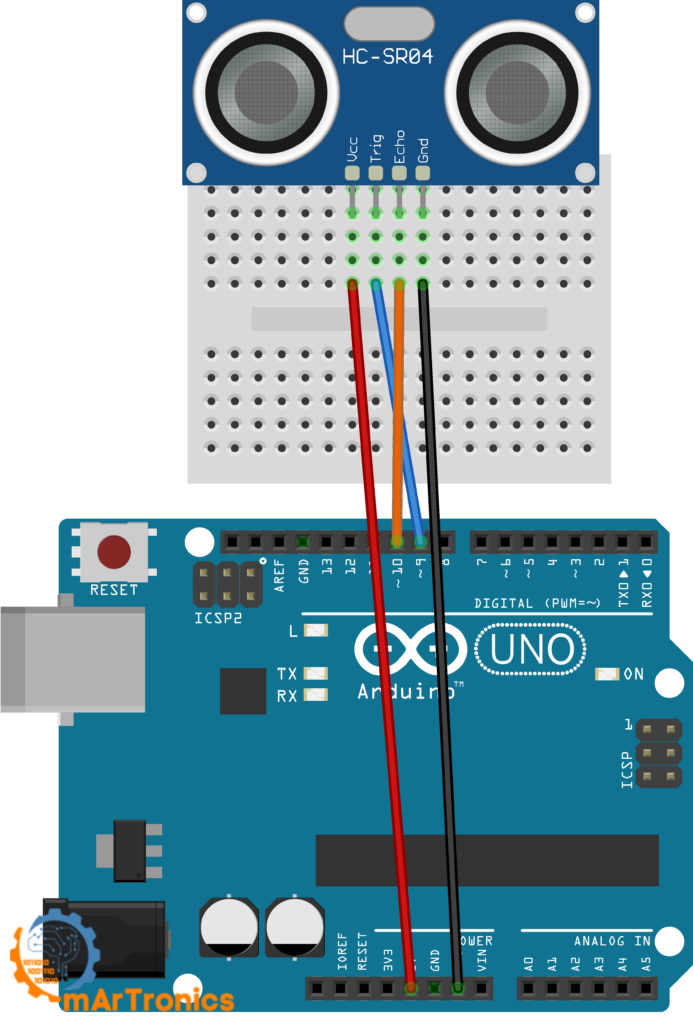
Programming the Arduino
Once everything is connected, you’ll need to program the Arduino to read the signals from the ultrasonic sensor:
/** * Author: Omar Draidrya * Date: 2024/05/07 * This code measures distance using an ultrasonic sensor and outputs the result via serial communication. */ #define TRIG_PIN 9 // Trigger pin of the ultrasonic sensor #define ECHO_PIN 10 // Echo pin of the ultrasonic sensor void setup() { pinMode(TRIG_PIN, OUTPUT); // Set the TRIG_PIN as an Output pinMode(ECHO_PIN, INPUT); // Set the ECHO_PIN as an Input Serial.begin(9600); // Start the serial communication } void loop() { long duration, distance; digitalWrite(TRIG_PIN, LOW); // Clear the TRIG_PIN delayMicroseconds(2); // Wait for 2 microseconds digitalWrite(TRIG_PIN, HIGH); // Set the TRIG_PIN high delayMicroseconds(10); // Wait for 10 microseconds digitalWrite(TRIG_PIN, LOW); // Set the TRIG_PIN low duration = pulseIn(ECHO_PIN, HIGH); // Read the ECHO_PIN and return the duration of the pulse distance = duration * 0.034 / 2; // Calculate the distance in cm Serial.print("Distance (cm): "); // Print "Distance (cm): " to the serial monitor Serial.println(distance); // Print the distance to the serial monitor delay(1000); // Wait for 1 second before next measurement }
This sketch sets up the Arduino to trigger the ultrasonic sensor and then listen for the echo. The pulseIn() function measures the length of the incoming pulse, which is then converted to a distance using the speed of sound.
Connecting an HD44780 16x2 LCD Display with an I2C Serial Adapter to an Arduino for Ultrasonic Distance Measurement
Displaying distance measurements from an ultrasonic sensor on an LCD screen enhances the usability and functionality of your Arduino project. In this guide, we will connect an HD44780 16×2 LCD display equipped with an I2C serial adapter to an Arduino, alongside an HC-SR04 ultrasonic sensor. This setup simplifies the wiring and coding process, allowing the display of real-time distance measurements.
Hardware Setup
To connect your LCD display with the I2C adapter and the HC-SR04 ultrasonic sensor to the Arduino, follow these connections:
- LCD Display with I2C Adapter:
– VCC to Arduino 5V: Powers the LCD.
– GND to Arduino GND: Common ground.
– SDA to Arduino A4 (SDA): Data line for I2C communication.
– SCL to Arduino A5 (SCL): Clock line for I2C communication. - HC-SR04 Ultrasonic Sensor:
– VCC to Arduino 5V: Powers the sensor.
– GND to Arduino GND: Common ground.
– TRIG to Arduino Pin 9: Trigger pin to send the pulse.
– ECHO to Arduino Pin 10: Echo pin to receive the pulse.
Ensure all connections are secure on your breadboard or through direct wiring before proceeding.
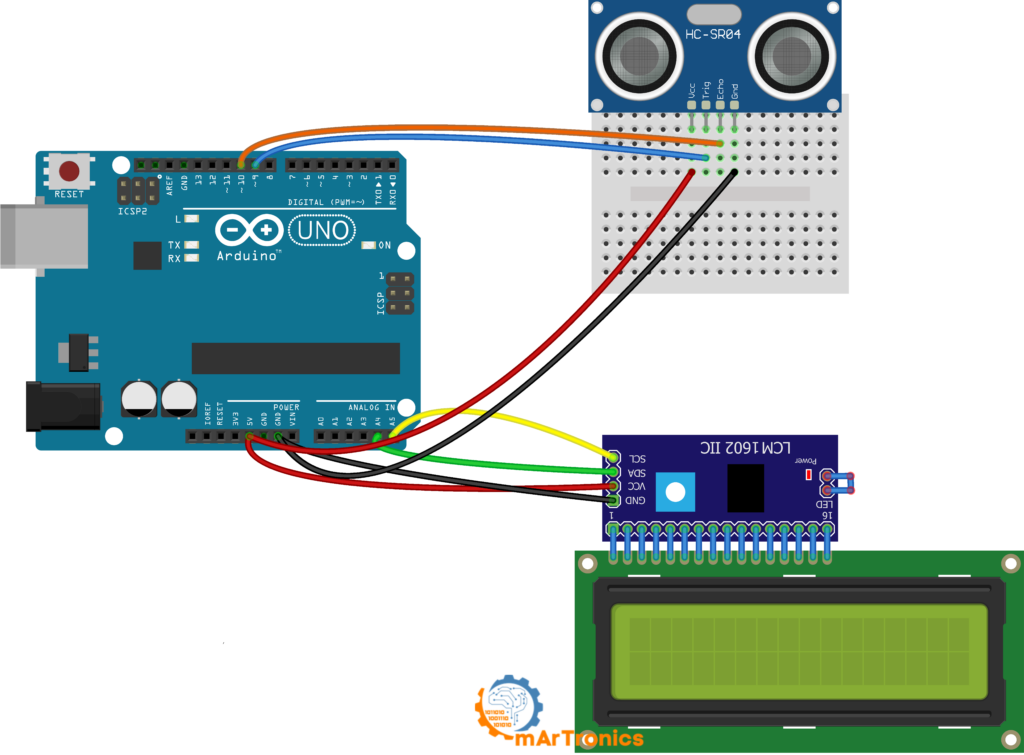
Arduino Code
The following Arduino sketch initializes both the ultrasonic sensor and the LCD display, then continuously measures the distance and displays it on the LCD. The sketch uses the NewPing library for the ultrasonic sensor and the LiquidCrystal_I2C library for the LCD, both of which need to be installed via the Arduino Library Manager before uploading the sketch.
/** * Author: Omar Draidrya * Date: 2024/05/07 * This code measures distance using an ultrasonic sensor and displays the result on an LCD. */ #include <LiquidCrystal_I2C.h> #include <NewPing.h> #define TRIGGER_PIN 9 // Trigger pin of the ultrasonic sensor #define ECHO_PIN 10 // Echo pin of the ultrasonic sensor #define MAX_DISTANCE 200 // Maximum distance for ping (in centimeters) LiquidCrystal_I2C lcd(0x27, 16, 2); // Set the LCD address to 0x27 for a 16x2 display NewPing sonar(TRIGGER_PIN, ECHO_PIN, MAX_DISTANCE); // Initialize the ultrasonic sensor void setup() { lcd.init(); // Initialize the LCD lcd.backlight(); // Turn on the backlight lcd.clear(); // Clear the display lcd.setCursor(0, 0); // Set the cursor to the first column, first row lcd.print("Distance:"); // Print static text } void loop() { delay(50); // Delay between measurements int distance = sonar.ping_cm();// Get distance in cm lcd.setCursor(0, 1); // Move cursor to the second row if (distance > 0) { // Check if the distance is greater than zero lcd.print(distance); // Display the distance lcd.print(" cm "); // Print units and clear extra characters } else { lcd.print("Out of range "); // Display out of range } }
This setup efficiently displays distance measurements on an LCD, providing a clear and user-friendly output for your ultrasonic distance measuring project. The integration of the I2C adapter with the LCD minimizes the wiring complexity and saves valuable I/O pins on the Arduino, making it ideal for projects with multiple sensors or inputs. This makes it perfect for educational purposes, DIY projects, or any application needing distance feedback.
Applications and further research
With this setup, you could integrate the ultrasonic sensor into a variety of projects, such as
Robotics: Avoiding obstacles or navigating paths.
Security systems: Detecting movement or breaches in a defined area.
Automated measurement tools: For industries requiring accurate spatial measurements.
Conclusion
Integrating an ultrasonic sensor with an Arduino opens up a wealth of possibilities for creating interactive and responsive projects. Whether you’re building a smart robot, designing a parking sensor, or experimenting with new sensing technologies, the HC-SR04 provides a reliable and simple option for distance measurement and environmental sensing. Have fun exploring the possibilities of ultrasonic technology in your projects!